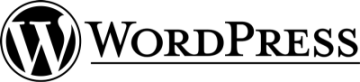
Having non-standard table prefixes can help reduce automated attacks and malicious scripts from compromising your WordPresss database
This is a fairly simple process if you are familiar with the underlying database and principles, but is not recommended unless you are experienced with queries and a sql client such as phpMyAdmin
1) Prepare by making a backup everything, redirect your visitors etc
2) Change the default table prefix in wp-config.php to your required name
3) Rename all WordPress database table prefixes
Here are the necessary SQL commands (remember to change the example to your own table prefix)
RENAME table 'wp_commentmeta' TO 'wp_rand0mstr1ng_commentmeta';
RENAME table 'wp_comments' TO 'wp_rand0mstr1ng_comments';
RENAME table 'wp_links' TO 'wp_rand0mstr1ng_links';
RENAME table 'wp_options' TO 'wp_rand0mstr1ng_options';
RENAME table 'wp_postmeta' TO 'wp_rand0mstr1ng_postmeta';
RENAME table 'wp_posts' TO 'wp_rand0mstr1ng_posts';
RENAME table 'wp_terms' TO 'wp_rand0mstr1ng_terms';
RENAME table 'wp_term_relationships' TO 'wp_rand0mstr1ng_term_relationships';
RENAME table 'wp_term_taxonomy' TO 'wp_rand0mstr1ng_term_taxonomy';
RENAME table 'wp_usermeta' TO 'wp_rand0mstr1ng_usermeta';
RENAME table 'wp_users' TO 'wp_rand0mstr1ng_users';
Remember if there are other WordPress related tables created by plugins you will need to rename these as well. ALL table prefixes should be renamed.
4) Search the options table for any instances of the old table prefixes
SELECT * FROM 'wp_rand0mstr1ng_options' WHERE 'option_name' LIKE '%wp_%'
This will return wp_user_roles and any options or configurations created by plugins or custom scripts. Update the fields as appropriate.
5) Edit the usermeta table
Search the usermeta for all instances of the old table prefixes
SELECT * FROM 'wp_rand0mstr1ng_usermeta' WHERE 'meta_key' LIKE '%wp_%'
Again these fields will need to be updated where appropriate
Test everything is working and then set your site to live once again.